Unity 개발을 하다 보면 GetComponent를 빈번하게 사용하게 됩니다. 하지만 매번 호출할 때마다 성능 오버헤드가 발생한다는 사실을 알고 계신가요? 이번 포스팅에서는 GetComponent를 캐싱하여 최적화하는 방법과 그 중요성에 대해 다뤄보겠습니다.
캐싱(Caching)이란?
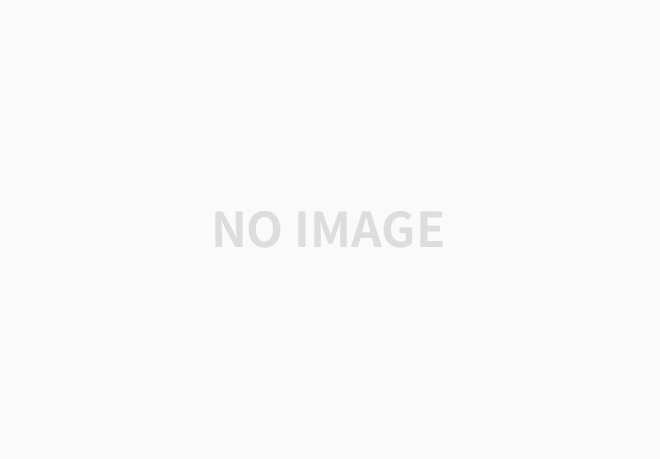
캐싱: 자주 사용되는 데이터를 미리 저장해두고, 이후에 해당 데이터에 빠르게 접근할 수 있도록 하는 기술
캐싱은 성능을 크게 향싱시키는 중요한 최적화 기법 중 하나
특히 GetComponent와 같은 무거운 연산을 반복적으로 호출하는 상황에서 매우 유용함
이제 캐싱으로 GetCompoent를 최적화하는 방법을 살펴보자
CachedMonobehaviour
using System;
using System.Collections.Generic;
using UnityEngine;
namespace DevelopKit.System
{
public class CachedMonobehaviour : MonoBehaviour
{
private Dictionary<Type, Component> _cachedComponents = new Dictionary<Type, Component>();
protected T GetCachedComponent<T>() where T : Component
{
Type type = typeof(T);
if (_cachedComponents.ContainsKey(type))
{
return (T)_cachedComponents[type];
}
T component = GetComponent<T>();
if (component != null)
{
_cachedComponents[type] = component;
}
return component;
}
private Transform _cachedTransform;
public new Transform transform
{
get
{
if (_cachedTransform == null)
{
_cachedTransform = base.transform;
}
return _cachedTransform;
}
}
}
}
사용 방법
1. Unity 프로젝트에 CachedMonobehaviour.cs 파일 다운로드 및 추가
2. GetComponent 함수가 아니라, GetCachedComponent 함수를 사용
코드 원리
위 코드를 살펴보면 _cachedComponents 필드를 확인할 수 있는데, 이 필드가 캐시의 역할을 수행함
GetCachedComponent 함수를 호출하면 _cachedCompoents에 캐싱된 적이 있는지 체크함
만약 있다면 저장된 캐시를 반환하고, 아니라면 GetCompoent 함수를 수행하여 값을 반환함
(이때, _cachedCompoents에 값을 캐싱하는 작업을 수행해야 함)
💡 사용 시 유의할 점
캐싱을 사용하면 성능을 향상시킬 수 있지만 추가적인 메모리를 필요로 함
그렇기 때문에 GetComponent를 자주 쓰는 경우에만 CachedMonobehaviour을 사용하여 최적화하는 것이 좋음
참고 자료
https://drehzr.tistory.com/2327
Unity) MonoBehaviour Base (자주사용하는 Component Cache)
MonoBehaviour Base (자주사용하는 Component Cache) using UnityEngine;using System.Collections.Generic;public class BaseMonoBehaviour : MonoBehaviour{ // 컴포넌트를 캐싱할 딕셔너리 private Dictionary _componentCache = new Dictionary(
drehzr.tistory.com
'유니티(Unity) > 콘텐츠 기능' 카테고리의 다른 글
[Unity 기능] 2D Endless Platform 구현 (2편) (0) | 2025.01.02 |
---|---|
[Unity 기능] 2D Endless Platform 구현 (1편) (2) | 2025.01.01 |
[Unity 기능] 컴파일 시점 제어하기 (1) | 2024.12.25 |
[Unity 기능] Sprite Atlas에 대하여 (1) | 2024.12.15 |
[Unity 기능] 9-Slicing Sprite 제작 (1) | 2024.12.07 |